Linux Inter Process Communication (Ipc) From Scratch In C
Linux Inter Process Communication (Ipc) From Scratch In C Last updated 7/2022
MP4 | Video: h264, 1280x720 | Audio: AAC, 44.1 KHz
Language: English
| Size: 5.80 GB[/center]
| Duration: 8h 46m
Linux Course - Includes Socket Programming, Linux System Programming, C programming - From Beginner to Expert
What you'll learn You will be able to Design Application which require IPC
Choose the best IPC mechanism depending on the application requirement
Understand the Linux IPC programming interface
Understand Linux OS better and feel confident
Prepare for IPC based interview Questions
Design a Linux process which could harness benefits of various IPC Mechanism at the same time
Requirements Basic C is essential
Basic knowledge Operating System shall be good
Zeal and Enthusiasm to learn
Description About This Course is about learning How Linux Processes Talk to each Other. This is a sub-domain of Linux System Programming. We shall explore various popular mechanism used in the industry through which Linux processes to exchange data with each other. We will go through the concepts in detail behind each IPC mechanism, discuss the implementation, and design and analyze the situation where the given IPC is preferred over others.We also discuss how applications should be designed to use IPC facilities provided by underlying Linux OS.You will have Assignments wherever possible, and throughout the course, there shall be one project in which you shall be incrementally applying the new IPC technique you have learned. Towards the end of the course, you would have practiced and applied all IPC techniques learned in this course.Each IPC mechanism, we will have a detailed code walk in which I show you how actually a given IPC mechanism is implemented on sending and receiving side. When you would join the industry, from day 1 you will witness IPC concepts being applied all over the software in order to facilitate communication between different parts of the software.Who should do this course ?This course is meant for UG Computer science students, job seekers, and professional developers. This is a MUST do course for those who want to join MNCs as a developer in System Programming. In System Programming, almost all the time you have to use IPC to carry out data exchange between processes, therefore students graduating in computer science and looking to seek an opportunity in MNCs as a developer should have IPC concepts at his/her fingertips.Pre-requisiteIt shall be advantageous if you know a little about C and OS. We designed this course assuming the student is a complete beginner in Linux IPC and we raise the level of course gradually as we move from Basic to advance concepts wherever necessary.Also, please just do not sit and watch my codes. Write your own codes, even if it is the same as mine!Related CoursesRPC (Remote Procedure Calls) is another way of carrying out Inter-Process Communication between two processes running on separate machines in the network. You may also want to check my other course on Linux RPCs where you will learn how to implement RPCs from scratch.Programming Language used In this course :We have strong reasons to choose C as a language for this course:IPC is a facility provided by the OS to developers to carry out data exchange between processes. Learning IPC using C helps you understand what is going on behind the scenes. C language really exposes the low-level details about how the system actually works. In System programming, C is the only language to be used and there is not even a remote substitute of this language when it comes to System programming.No Third-Party librariesWhatever logic you implement, you need to implement it from scratch. This course does not suggest taking the help of any third party library to get the jobs done. Use of external libraries completely defeats the purpose of the course. However, it is recommended to use third-party libraries for commonly used data structures such as linked lists/Trees/Queues, etc which saves a lot of time implementing these data structures.Note1 : Though we use Linux to teach the IPC techniques, conceptually, IPC of Linux is not very different from other OS platforms such as windows, iOS, etc. So, if you are a programmer for other platforms, this course still holds great value for you.Note2 : This Course talks about various techniques regarding exchanging data between processes, the other related topics such as process synchronization and locking is out of the scope of this course and will be covered separately.Warning: This course has auto system-generated subtitles which may not be perfect. Please disable subtitles as per your convenience.CurriculumIntroductionTable of ContentsComputer Architecture - OverviewVarious IPC TechniquesCommunication TypesIPC Technique 1 - Unix Domain SocketsSocket API IntroductionSocket Message TypesSocket Design ParadigmAccept System CallIntroducing Unix Domain SocketUnix Domain Socket Server ImplementationUnix Domain Socket Client ImplementationMultiplexingSelect System CallMultiplexing Server State machineMultiplexed Server ImplementationData Synchronization - IPC Project part 1IPC Technique 2 - Message QueueIntroductionMsgQ as a Kernel ResourceOpen & Create a MsgQClosing a MsgQEnque Data in MsgQDequeue Data from MsgQUnlinking a MsgQUsing a Msg Q - Design perspectiveBi-Directional Communication Code Walk - ImplementationDemonstrationIPC Technique 3 - Shared MemoryOverall Design GoalsConcept of Virtual Memory Program Control BlockShared Memory BasicsKernel Memorymmap() - Memory MappingDesign ConstraintShared Memory related APIsData Synchronization - IPC Project part 2IPC Technique 4 - SignalsIntroductionLinux Well knows SignalsSignals Generation and TrappingSending Signals using Kill()Data Synchronization - IPC Project part 3IPC Technique 5 - Network socketsSocket Programming DesignSelect System callAccept System callConcept of MultiplexingServer State machineProject on Socket Programming - IPC Project 4Multiplexing on Different IPCsUse select() to multiplex on different IPC interfaces
Overview
Section 1: Table of Contents
Lecture 1 Table of Contents
Lecture 2 Join Telegram Group
Lecture 3 Introduction
Section 2: Pre-requisites - Setting up Linux Development Environment
Lecture 4 Setting up Linux Development Environment
Section 3: IPC Technique 1 - Unix Domain Sockets
Lecture 5 Sockets Introduction
Lecture 6 Socket Message Types
Lecture 7 Socket Design
Lecture 8 Socket Accept() System Call
Lecture 9 Unix Domain Socket Introduction
Lecture 10 Unix Domain Server Implementation part 1
Lecture 11 Unix Domain Server Implementation part 2
Lecture 12 Unix Domain Client Implementation
Lecture 13 Unix Domain Client Server Demonstration
Lecture 14 Unix Domain IPC - Summary and observation
Lecture 15 Concept of Multiplexing
Lecture 16 Understanding select() System Call
Lecture 17 Multiplexing State Machine
Lecture 18 Multiplexed Unix Domain Server Implementation
Lecture 19 Multiplexed Unix Domain Server Demonstration
Lecture 20 Project - Data Synchronization using Unix Domain Sockets as an IPC
Section 4: IPC Technique 2 - Message Queues
Lecture 21 Introduction
Lecture 22 MsgQ as a kernel Resource
Lecture 23 Opening and Creating a MsgQ
Lecture 24 Closing a MsgQ
Lecture 25 Enque A Msg into MsgQ
Lecture 26 Deque a Msg from a MsgQ
Lecture 27 Unlink a MsgQ
Lecture 28 How to Use a MsgQ as an IPC
Lecture 29 Code Walk and Implementation
Lecture 30 Demonstration of msgQ as an IPC
Section 5: IPC Technique 3 - Shared Memory
Lecture 31 Agenda
Lecture 32 Paging Recap And Memory Mapping
Lecture 33 How Memory Mapping Works
Lecture 34 Shared Memory
Lecture 35 Using RAM as Shared Memory
Lecture 36 Example Codes
Lecture 37 Get familiar with mmap()
Lecture 38 Demo Program - Map Text File in Process Virtual Memory
Lecture 39 Design Constraints for using SHM as IPC
Lecture 40 Project - Data Synchronization using Shared Memory as an IPC
Section 6: IPC Technique 4 - Signals
Lecture 41 Signals Introduction
Lecture 42 Linux Well Known Signals
Lecture 43 Linux Signal Generation and Signal Trapping
Lecture 44 Sending Signal using kill
Lecture 45 Project - Data Synchronization using Signals as an IPC
Section 7: Network Socket Programming
Lecture 46 Disclaimer
Lecture 47 Introduction to Socket Programming
Lecture 48 Server Designing
Lecture 49 Accept system call
Lecture 50 Select System Call
Lecture 51 Implementing Multiplexing with Accept & Select System Calls
Lecture 52 TCP Server Example - part 1
Lecture 53 TCP Server Example - part 2
Lecture 54 TCP Server Example - part 3
Lecture 55 TCP Server Design Observation
Lecture 56 TCP Client Design and Implementation
Lecture 57 TCP Server Client Demonstration
Lecture 58 TCP Server With Multiplexing - High Level Design - Part1
Lecture 59 TCP Server With Multiplexing - Implementation - part 2
Lecture 60 TCP Server With Multiplexing - Demonstration - part 3
Lecture 61 Socket Programming Conclusion
Lecture 62 Project on TCP Server Designing Programming
Section 8: Multiplexing on Multiple IPCs
Lecture 63 Multiplexing on Multiple IPCs
Section 9: Project on Socket Programming
Lecture 64 Project Goals
Lecture 65 Project Deployment
Lecture 66 PUT Request Algorithm
Lecture 67 GET Request Algorithm
Lecture 68 Message Types
Lecture 69 Messages Processing
Lecture 70 Projects Pseudocode
Lecture 71 Final Words before Coding
Lecture 72 Bonus
Under graduate Computer Science Students,Post Graduate Students,Job Seekers in System programming Domain - Networking/Driver programming/Distributed Systems/IOT etc
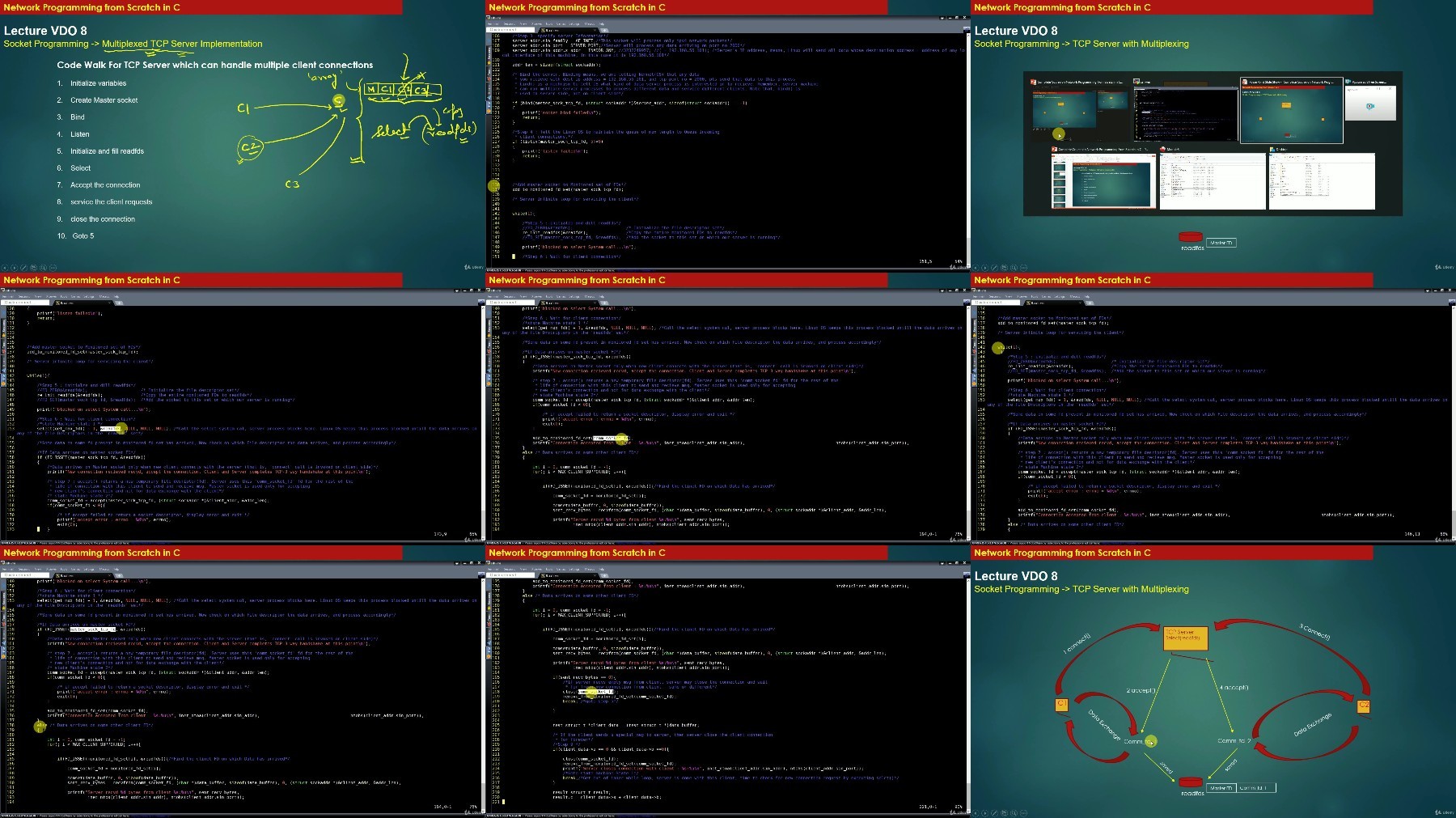

Free search engine download: Udemy Linux Inter Process Communication IPC from Scratch in C 2022-7